Want to build a UI component library that saves time, reduces inconsistencies, and ensures accessibility? Here’s what you need to know upfront:
- Key Components: Buttons, grids, modals, and more – all designed with accessibility (WCAG 2.2 AA) and scalability in mind.
- Design Standards: Use consistent typography, color tokens, and responsive breakpoints to create visually aligned components.
- Accessibility: Implement ARIA roles, proper contrast ratios, and screen reader compatibility for inclusive designs.
- Version Control: Use semantic versioning (SemVer) to manage updates and track changes.
- Testing: Cover accessibility, visual regression, and component behavior with tools like axe-core, Percy, and Jest.
- Integration: Sync with design tools (like Figma or UXPin) and use CSS variables for dynamic theming.
Table of Contents
Accessibility Flavored React Components Make your Design System Delicious
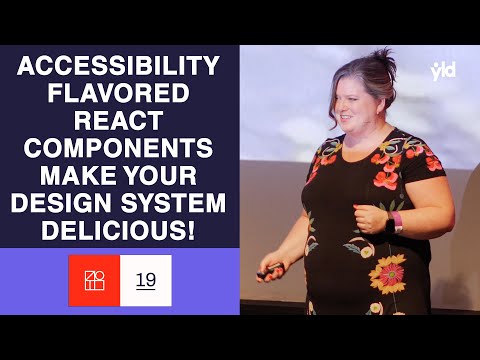
Must-Have UI Components
These essential components tackle the inconsistency issues mentioned earlier while meeting the scalability needs discussed later. Nearly all modern applications (98%) rely on these fundamental elements .
Buttons and Input Controls
When designing buttons and input controls, focus on features like:
- Text fields with built-in validation
- Dropdown menus that support single and multi-select options
- Checkboxes and radio groups for selecting from multiple options
- Toggle switches for binary choices
- Search bars with autocomplete for better usability
MUI Base sets a strong example here with its WCAG-compliant focus ring implementations , ensuring clear visual feedback across all devices.
Page Layout Components
A strong layout system is non-negotiable. Material UI‘s Grid system is a standout, offering breakpoint-based responsiveness . To maintain uniformity, use an 8px base unit for spacing. This approach ensures consistent layouts and smooth multi-device experiences, as outlined in the design standards.
Key layout components include:
- Responsive grid systems for adaptable designs
- Flex containers for arranging dynamic content
- Card components with consistent spacing guidelines
- Data tables equipped with sorting, pagination, and accessibility features
These elements form the backbone of a reliable and scalable UI framework.
User Status Components
Status components are crucial for providing feedback and guiding users. Focus on:
- Modal dialogs with full keyboard navigation
- Toast notifications using aria-live regions for accessibility
- Progress indicators to show loading states
- Error banners with high contrast for visibility
- Empty state placeholders to handle data views gracefully
Radzen‘s WCAG-compliant solutions are a great reference, featuring 48px minimum touch targets to ensure usability on any device. These components are vital for creating accessible and user-friendly interfaces.
Design Standards
Expanding on the core components mentioned earlier, these guidelines focus on achieving both visual consistency and technical reliability. Angular Material, for example, uses CSS variables for primary, accent, and warning colors , making it easier to manage themes without complicated CSS overrides.
Color and Typography Rules
Use CSS variables for colors and typography to meet WCAG 4.5:1 contrast ratios . Consistent typography scales help avoid layout issues, which affect 78% of design teams . Establish a clear hierarchy with relative units for better readability and structure:
Element Type | Size (rem) | Usage Context |
---|---|---|
Page Titles | 2.5 | Main headers |
Card Headers | 1.75 | Section titles |
Body Text | 1 | Regular content |
Pair heading and body fonts with consistent line heights to maintain a balanced look. Tools like Figma Style Libraries can ensure design and development stay aligned . These tokenized systems also address accessibility needs, which are covered in later sections.
Component States
Every component should define six key interactive states. MUI Base offers a strong example by using :focus-visible pseudo-classes to manage focus rings . The essential states include:
- Default: The component’s standard appearance.
- Hover: Visual feedback for mouse interaction.
- Active: Indicates a pressed or selected state.
- Focus: Highlights keyboard navigation.
- Disabled: Shows the component is unavailable.
- Error: Signals invalid input or a problem.
To enhance user experience, keep hover state transitions smooth with a duration of 300ms.
Multi-Device Support
Modern component libraries need to work effortlessly across different devices. CoreUI demonstrates this well with adaptive grid layouts that shift from vertical stacks on mobile to horizontal arrangements on desktops .
Responsive Breakpoints:
Breakpoint | Width |
---|---|
Mobile | 320px |
Tablet | 768px |
Desktop | 1024px |
These breakpoints align with the grid systems discussed in Must-Have Components. Use CSS container queries for more flexible, component-specific adjustments. Additionally, CSS clamp() can help achieve fluid scaling for typography and spacing across various screen sizes.
sbb-itb-f6354c6
Accessibility Standards
Along with maintaining a consistent visual design, accessibility standards ensure components work effectively for everyone. This approach not only supports usability but also addresses the previously mentioned 25% reduction in maintenance costs. By adhering to these standards, components can be reused across different projects while reducing legal risks – both critical for the long-term success of any component library.
Creating accessible component libraries requires careful attention to navigation and technical details.
Navigation Support
Use WAI-ARIA 1.2 patterns to enable smooth keyboard navigation, such as arrow key menu movement and activating elements with the Enter key . Focus management should rely on semantic HTML elements, as seen in Angular Material .
Keyboard Action | Expected Behavior |
---|---|
Tab Key | Moves between interactive elements |
Arrow Keys | Navigates within a component |
Enter/Space | Activates the current element |
Escape | Closes or cancels the action |
Technical Requirements
Ensure compatibility with assistive technologies by implementing ARIA roles and attributes. For example, CoreUI for Vue uses a .visually-hidden
CSS class to hide decorative elements while maintaining accessibility .
Key technical practices include:
-
ARIA Roles and Attributes: Use
role="dialog"
andaria-modal="true"
for modals. Addaria-invalid
andaria-describedby
to form fields to indicate error states . - Contrast Standards: Adhere to WCAG 2.1 AA guidelines, ensuring a 4.5:1 contrast ratio for regular text and 3:1 for larger text. Automated tools can check contrast levels during the build process.
- Screen Reader Compatibility: Incorporate semantic HTML and proper ARIA labels. While MUI Base emphasizes accessibility, it also notes that a component library alone can’t guarantee full application compliance .
Testing with screen readers like NVDA and JAWS helps confirm consistent behavior across platforms.
Additionally, component documentation should include an accessibility section with implementation examples. Angular Material sets a strong example by offering keyboard interaction diagrams and detailed ARIA role guidelines for each component .
Growth and Tool Integration
Once quality controls are in place, the next step is to focus on building scalable infrastructure. This can be achieved by addressing three key areas: version control, theming, and design tool integration. Tools like Turborepo simplify cross-project updates while ensuring dependencies remain intact.
Version Control
Version control is more than just using Git. Implementing semantic versioning (SemVer) helps teams systematically track updates and breaking changes. For example, MUI Base uses SemVer alongside component-specific changelogs .
Version Type | When to Use | Example Change |
---|---|---|
Major (1.0.0) | For breaking changes | Prop API restructure |
Minor (0.1.0) | For new features | Adding new variants |
Patch (0.0.1) | For bug fixes | Fixing style issues |
This structured approach ensures workflows stay aligned and avoids the confusion caused by untracked changes.
Theme System
A strong theme system is essential for supporting multiple brands while adhering to accessibility standards. By combining CSS custom properties with design tokens, teams can create a foundation for dynamic and adaptable styling. For instance, MUI Base uses a provider pattern to manage themes:
const theme = {
colors: {
primary: 'var(--primary-color, #1976d2)',
secondary: 'var(--secondary-color, #dc004e)'
}
}
Using CSS variables allows for runtime theme switching while maintaining WCAG contrast ratios . Teams can create presets that override base variables without affecting component functionality. This approach ensures scalability across multiple brands while keeping accessibility intact.
Design Software Setup
For component libraries, syncing code with design tools is crucial to maintain consistency. Integrations like UXPin’s Merge allow designers to work directly with production-ready components in their design environment. This bridges the gap between design and development, supporting the 60% reduction in inconsistencies mentioned earlier.
Key integration practices include:
- Synchronizing tokens via Style Dictionary
- Updating components through Storybook
- Using Figma Dev Mode annotations to reflect code props
Quality Control
Expanding on version control and theme systems discussed earlier, maintaining consistent quality is key as libraries grow. This ensures components remain reliable at scale.
Automated Tests
Use a multi-layered testing approach to cover every aspect of your components. Key types include visual regression, accessibility, unit, and integration tests:
Test Type | Tool | Focus Areas |
---|---|---|
Visual Regression | Percy/Chromatic | Looks of components, responsive design |
Accessibility | axe-core | WCAG compliance, ARIA attributes |
Unit Testing | Jest | Component behavior |
Integration Testing | Cypress | Interactions between components |
For example, Atlassian’s team identifies around 15 visual regressions weekly before production. These tests also ensure adherence to accessibility standards discussed earlier.
Component Updates
Updating components effectively requires a clear and structured strategy. Semantic versioning paired with deprecation notices provides transparency and eases transitions:
// Example of a deprecation notice
if (process.env.NODE_ENV !== 'production') {
console.warn(
'ButtonLegacy will be removed in version 2.0.0. ' +
'Please migrate to the Button component.'
);
}
This approach minimizes disruption while encouraging teams to adopt updated components.
Usage Tracking
Tracking metrics helps teams assess component performance and adoption. Focus on:
- Adoption rates: Aim for at least 80% usage of core components.
- Customization levels: Flag components when customizations exceed 15%.
- Error monitoring: Use tools like Sentry to track issues.
- Documentation metrics: Measure engagement with guides and examples.
Monitoring customization levels helps prevent workflow misalignment, as noted earlier. Integrating error tracking into your CI/CD pipeline ensures problems are identified and resolved early, keeping quality consistent across versions.
Conclusion
UI component libraries can achieve over 70% component reuse and maintain full WCAG compliance by following this checklist . This structured approach has been shown to reduce inconsistencies by 60% and cut maintenance costs by 25%, as noted earlier.
When applying this checklist, focus on measurable results like documentation engagement (over 2 minutes per page) and style consistency (less than 5% variance). These metrics can be tracked using the quality controls discussed earlier. A versioned rollout strategy has also proven critical for ensuring steady growth.