How to Run React App Locally – A Step-by-Step Guide
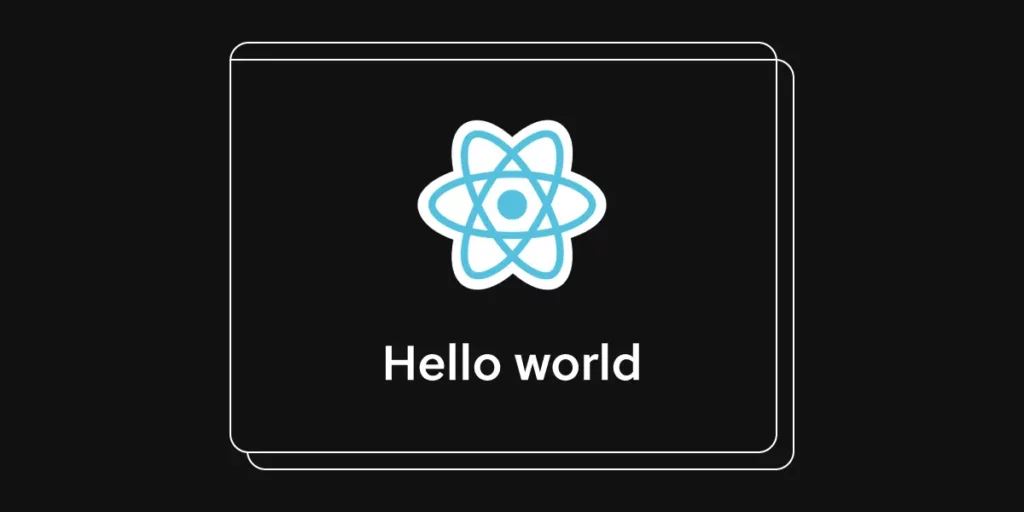
Are you ready to dive into the world of React but not sure how to get your local application up and running? Well, you’ve clicked on the right article! Whether you’re a budding frontend developer eager to showcase your newly acquired React skills or simply someone curious about what it takes to launch a local React application, this step-by-step guide is tailored just for you.
In this comprehensive walkthrough, we’ll start from the very basics and work our way up to launching your first React application on your local machine. By the end of this guide, you’ll have a solid understanding and hands-on experience with React. So, let’s get the ball rolling.
Need to build a React UI? You’re in the right place! UXPin Merge is a UI builder that allows you to drag and drop functional React components from MUI, Ant design, and any other library you want, adjust the properties and copy the code to your development environment. Try UXPin Merge for free.
A quick note about React for beginners
Learning to code might feel like trekking through a jungle if you’re new to it. React, a wildly popular JavaScript library for building user interfaces, is one of the clearings in this jungle. It lets developers create large web applications that can change data without reloading the page.
Why does this matter? React’s efficiency and flexibility make building interactive and dynamic web applications a smoother process. This guide requires a basic understanding of HTML, CSS, and JavaScript. If you’re not there yet, no worries—consider this an opportunity to dip your toes in!
What are local environments?
In web development, “local environments” refer to the setup and configuration of software and tools on a developer’s local computer to mimic a production server or hosting environment. This allows developers to build, test, and debug their applications in a controlled setting before deploying them to a live server.
Why would frontend developers run React apps locally?
By taking advantage of local environments, developers can ensure that their applications are robust, performant, and ready for deployment.
- During initial development – when starting a new project or feature, running locally allows you to quickly iterate and refine your code. Alternatively, you can use UXPin Merge to create a React app UI for this purpose.
- While testing new features – before merging new features or changes into the main codebase, you should test them locally to ensure they work as expected.
- For debugging issues – when bugs are identified, debugging locally allows you to use sophisticated tools and techniques to diagnose and fix issues efficiently.
- When learning and experimenting – if you’re learning React or trying out new libraries, doing so locally provides a safe and controlled environment to experiment without affecting existing projects.
- During code reviews and pair programming – running the app locally can facilitate code reviews and pair programming sessions, allowing for immediate feedback and collaborative problem-solving.
- For performance testing – you can perform performance tests locally to optimize your app before deploying it to a staging or production environment.
Setting Up Your Development Environment
Installing Node.js and npm
Let’s start with the basics: ensuring Node.js and npm are on your computer. These package managers are the bread and butter of modern web development, allowing you to run JavaScript outside a web browser.
Run node -v
and npm -v
in your terminal to check. If not, head over to the Node.js website for a straightforward installation process. Don’t forget to double-check the installation by running those commands again.
Visual Studio Code (VS Code)
Why do so many developers stick with VS Code? It’s like having a Swiss Army knife for coding. It’s free, lightweight, and supports a plethora of extensions to make your coding life much easier. To get it, download from the official site and install it. Boot it up and explore extensions like ‘ESLint’, ‘Prettier’, and ‘Reactjs code snippets’ to turbocharge your React development process.
Understanding the Terminal
A terminal allows you to interact with your computer through commands. macOS and Linux typically come with a terminal application already installed. Windows users can use Command Prompt, PowerShell, or Windows Subsystem for Linux (WSL).
The terminal, a text-based interface for typing commands, can be daunting at first glance. But don’t worry, you only need a few commands to start. Learn how to cd
(change directories), mkdir
(create directories), and rm
(remove files and directories). Think of it as learning the basic spells before diving into wizardry!
Your First Steps into React project
Now that you have the pre-requisites set up, let’s start with the creation of your first React application.
Setting up your React app
Creating a React app from scratch is simplified thanks to create-react-app, a bootstrapping tool provided by Facebook. To create your app, open your terminal, navigate to the directory where you want your project to live, and run the following command:
npx create-react-app my-react-app
Replace my-react-app with whatever name you wish to give your new project. This command sets up a new React project with a good default configuration. After the installation is complete, navigate into your project folder:
cd my-react-app
Understanding your React project structure
Upon navigating into your project folder, you’ll see several files and directories. Here’s a quick rundown of the most important ones:
- node_modules/: This directory contains all the packages and their dependencies that your project needs, as installed by npm.
- public/: This folder holds assets such as images, the index.html file, and the favicon.
- src/: The source directory where you’ll spend most of your time coding. It contains the JavaScript, CSS, and images that make up your web app.
- package.json: This file lists the packages your project depends on and includes other metadata relevant to your project.
Running your React app locally
To see your React application in action, run the following command in your terminal:
npm start
This command starts a development server and opens up your default web browser to http://localhost:3000
, where you can see your new React app running. If everything is set up correctly, you should see the default React welcome page.
Seeing your React app live for the first time is like watching your code take its first breath—it’s exhilarating! To stop the server, a simple Ctrl + C
in your terminal will do the trick.
Customizing your React app
Now that your app is up and running let’s make some minor adjustments to personalize it.
Modifying the React Component
Open the src/App.j
s file in your code editor. You’ll see a function App()
that returns a piece of JSX code. JSX is a syntax extension for JavaScript, commonly used with React to describe what the UI should look like.
Replace the content of App.js
with the following code to create a simple greeting message:
jsx
function App() {
return (
<div className="App">
<header className="App-header">
<p>Hello, React Developers!</p>
</header>
</div>
);
}
export default App;
Save the file, and you should see the text “Hello, React Developers!” replace the React logo on the page served at http://localhost:3000
.
Managing Dependencies and Packages
npm and package.json
npm plays a pivotal role in the React ecosystem, managing the packages your project depends on. The package.json file is the heart of your project, listing these dependencies and other configurations. Adding a package is as simple as npm install package-name, and removing one is just npm uninstall package-name.
Dependencies vs DevDependencies
Understanding the distinction here is crucial. Dependencies are packages your app needs to run, like React itself. DevDependencies are tools you use during development, like code formatters or testing libraries. Choosing correctly keeps your app lean and mean.
Using .env for Environment Variables
Environment variables let you manage sensitive information and configurations outside your codebase, making your project more secure and adaptable. Setting up a .env file at your project root and accessing these variables in your React app is straightforward and keeps your secrets safe.
Making Your React Application Ready for Deployment
Optimizing the Build
Before your app can fly on the internet, creating a production build is essential. This optimizes your app for performance, making it faster and more efficient. Run npm run build, and React will bundle up your app, ready for the digital world.
Pre-deployment Checklist
Ensure your app looks and works great on all devices, check all functionalities, and hunt down any pesky broken links or errors. This is your last line of defense before showing your creation to the world.
Deploying to a Web Server
When you’re ready, it’s time to choose a home for your app. Platforms like Netlify, Vercel, and GitHub Pages offer straightforward deployment processes, often just a few clicks away. Follow their guides, and before you know it, your app will be live for anyone to visit!
Prototyping in React is like creating a mini-version of your app before building the real thing. Imagine you want to build a Lego castle. Instead of just starting without a plan, you first sketch out how you want it to look, and maybe you even make a tiny model of it. UXPin Merge is a React prototyping tool that will help you build an interface of your React app just as you would build a Lego project.
Where to go from this tutorial?
You’ve just learned about launching local React applicatiosn. While this is merely the tip of the iceberg, there are limitless possibilities ahead as you delve deeper into React. Here are some suggestions on where to go from here:
- Learn about React state and lifecycle methods: Understanding how to manage state, React best practices and the lifecycle of components is crucial in React development.
- Dive into routing with React Router: For applications with multiple views, you’ll want to learn about routing.
- Explore external APIs: Fetch data from external APIs to make your application dynamic and interactive.
- Build a React app prototype: Experiment with creating prototypes of React application that use coded components.
FAQs
Do I need any prior programming knowledge to follow this guide? A basic understanding of HTML, CSS, and JavaScript is helpful but not mandatory. There’s no time like the present to start learning!
How can I update my React application to the latest version?
Keeping your app up-to-date is crucial. Run npm outdated to check for updates and npm update to apply them. Don’t forget to check the official React documentation for any breaking changes.
What should I do if npm start does not work?
First, don’t panic. Check your terminal for error messages—they’re usually very informative. If you’re stuck, a quick online search or asking for help in a React community can work wonders.
Are there alternatives to VS Code for React development?
Absolutely! While VS Code is popular, other code editors like Sublime Text, Atom, and WebStorm are equally capable. It’s all about personal preference.
Can I use this guide to configure a React app in a different operating system?
Yes, this guide is designed to be OS-agnostic. Whether you’re on Windows, macOS, or Linux, you can follow these steps to run your local React application.
Build React UI with UXPin Merge
Launching a local React application is just the beginning of your journey into React development. With its component-based architecture, React opens the door to building interactive, stateful web applications with ease.
Remember, the key to mastering React or any new technology is consistency and practice. So, keep experimenting, keep building, and don’t be afraid to break things. That’s all part of the learning process. If you want to practice building your app UI, use UXPin Merge. It’s a drag-and-drop UI builder that allows you to design apps and websites with React components. Design, copy the code, and create React projects faster than ever. Try UXPin Merge.