Dynamic data binding is what makes React apps interactive and responsive. It ensures your UI updates automatically when the underlying data changes. Here’s what you need to know:
- One-Way Data Flow: Data flows from parent to child components, making it easier to track changes and debug.
- State and Props: React uses
useState
for managing component state andprops
for passing data down the component tree. - Controlled Components: Form inputs are tied directly to state, keeping the UI and data in sync.
- Performance Optimization: React minimizes unnecessary re-renders by updating only the changed components.
Quick Example:
function Parent() {
const [user, setUser] = useState({ name: 'Jane', email: 'jane@example.com' });
return <Child user={user} />;
}
function Child({ user }) {
return <div>{user.name} - {user.email}</div>;
}
React’s approach to data binding simplifies state management and improves performance, making it ideal for building scalable, interactive UIs.
Table of Contents
- Data Binding with Custom Hooks
- Using Context for Data Management
- UXPin for React Prototyping
Components & dynamic databinding in React JS | React JS …
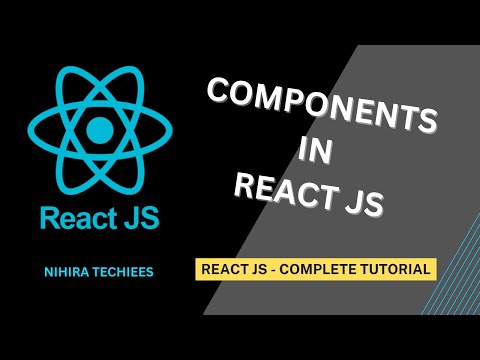
Data Binding Mechanics in React
React’s data binding approach ensures applications are predictable and easier to maintain. Let’s break down its key mechanisms with practical examples.
One-Way Data Flow Explained
React uses a one-way data flow model where:
- Data moves from parent components to child components through props.
- Parent components retain control over the data, preventing child components from making changes.
- React optimizes rendering by efficiently batching updates based on changes in data.
Using State and Props
State Management
- Components manage their internal state using the
useState
hook. - Only the component that owns the state can modify it.
- React schedules state updates intelligently to ensure performance stays optimal.
Props Flow
- Props allow data transfer from parent to child components.
- Props are read-only, meaning child components cannot modify them.
- If props change, React automatically re-renders the child component.
Here’s a simple example:
// Parent Component
function ParentComponent() {
const [userData, setUserData] = useState({
name: 'John',
email: 'john@example.com'
});
return <UserProfile user={userData} />;
}
// Child Component
function UserProfile({ user }) {
return (
<div>
<h2>{user.name}</h2>
<p>{user.email}</p>
</div>
);
}
This demonstrates how parent-to-child data flow works seamlessly in React.
Form Controls and Event Handling
Controlled components in React bind form inputs directly to state, making the React state the single source of truth. This setup ensures instant synchronization between user interactions and the UI.
function LoginForm() {
const [formData, setFormData] = useState({
username: '',
password: ''
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData(prevData => ({
...prevData,
[name]: value
}));
};
return (
<form>
<input
name="username"
value={formData.username}
onChange={handleChange}
/>
<input
name="password"
type="password"
value={formData.password}
onChange={handleChange}
/>
</form>
);
}
This example highlights how React keeps the UI and state in sync, ensuring a smooth user experience.
Building Data-Bound Components
Managing State with useState
Start managing state in your components using the useState
hook:
function UserProfile() {
const [profile, setProfile] = useState({
firstName: '',
lastName: '',
email: '',
phone: ''
});
const updateProfile = (field, value) => {
setProfile(prev => ({
...prev,
[field]: value
}));
};
return (
<div className="profile-form">
</div>
);
}
This approach lets you easily update specific fields in the state without affecting others.
Building Form Input Components
Reusable form components simplify data binding between the UI and your component’s state. Here’s an example:
function FormInput({ label, name, value, onChange }) {
return (
<div className="form-field">
<label htmlFor={name}>{label}</label>
<input
id={name}
name={name}
value={value}
onChange={e => onChange(name, e.target.value)}
className="form-input"
/>
</div>
);
}
You can use this component in a form like this:
function ProfileForm() {
const [formData, setFormData] = useState({
username: '',
email: ''
});
return (
<form>
<FormInput
label="Username"
name="username"
value={formData.username}
onChange={(name, value) => setFormData(prev => ({
...prev,
[name]: value
}))}
/>
<FormInput
label="Email"
name="email"
value={formData.email}
onChange={(name, value) => setFormData(prev => ({
...prev,
[name]: value
}))}
/>
</form>
);
}
This setup ensures that changes to each input field are reflected in the component’s state.
Handling Data Changes
Efficiently handle data changes by combining useState
with event handlers:
function ContactForm() {
const [formData, setFormData] = useState({
name: '',
email: '',
message: ''
});
const handleChange = useCallback((e) => {
const { name, value } = e.target;
setFormData(prev => ({
...prev,
[name]: value
}));
}, []);
const handleSubmit = useCallback((e) => {
e.preventDefault();
console.log('Form submitted:', formData);
}, [formData]);
return (
<form onSubmit={handleSubmit}>
</form>
);
}
Best Practices for Data-Bound Components
When building data-bound components, keep these tips in mind:
- State initialization: Use meaningful default values to avoid unexpected behavior.
- Optimize events: Use
useCallback
to prevent unnecessary re-renders. - Validate inputs: Implement error handling for form fields to improve usability.
- Improve performance: Batch state updates to minimize rendering overhead.
These practices will help create efficient, maintainable, and user-friendly components.
sbb-itb-f6354c6
Data Binding Guidelines
Leverage React’s data binding to streamline state management and component interactions. Here are some practical tips to get you started.
State Management in Parent Components
Keep your state centralized by lifting it to parent components. This ensures a single source of truth, especially when multiple child components depend on the same data:
function ParentComponent() {
const [sharedData, setSharedData] = useState({
userPreferences: {
theme: 'light',
fontSize: 16,
},
notifications: [],
});
const updatePreferences = (key, value) => {
setSharedData((prev) => ({
...prev,
userPreferences: {
...prev.userPreferences,
[key]: value,
},
}));
};
return (
<>
<PreferencesPanel
preferences={sharedData.userPreferences}
onUpdate={updatePreferences}
/>
<ContentDisplay
preferences={sharedData.userPreferences}
/>
</>
);
}
This structure ensures data updates are predictable and manageable.
Component Communication with Callbacks
Callbacks are essential for passing data updates from child components back to their parent:
function DataForm({ onDataUpdate, initialData }) {
const [formData, setFormData] = useState(initialData);
const handleFieldChange = (field, value) => {
const updatedData = {
...formData,
[field]: value,
};
setFormData(updatedData);
onDataUpdate(updatedData);
};
return (
<div className="form-container">
<FormField
name="username"
value={formData.username}
onChange={handleFieldChange}
/>
</div>
);
}
This pattern promotes a smooth flow of data between components.
Reducing Component Re-renders
Minimize unnecessary re-renders by splitting components and using memoization techniques:
const MemoizedInput = memo(function Input({ value, onChange }) {
return (
<input
value={value}
onChange={(e) => onChange(e.target.value)}
className="form-input"
/>
);
});
function DataBindingContainer() {
const [data, setData] = useState({ text: '' });
const handleChange = useCallback((value) => {
setData((prev) => ({
...prev,
text: value,
}));
}, []);
return (
<div className="container">
<MemoizedInput
value={data.text}
onChange={handleChange}
/>
<DataDisplay text={data.text} />
</div>
);
}
Here are some tips to keep your components efficient:
- Break down large components into smaller, focused pieces.
- Keep state close to where it’s used.
- Use
useMemo
to optimize expensive computations. - Stabilize functions with
useCallback
and wrap components withmemo
to avoid unnecessary updates.
These strategies will help you build a more efficient and maintainable UI.
Advanced Data Binding Methods
Building on the basics of state and event management, advanced techniques make data binding in React more efficient and reusable.
Data Binding with Custom Hooks
Custom hooks let you bundle complex data binding logic into reusable functions. Here’s an example of how to handle form data binding using a custom hook:
function useFormBinding(initialState) {
const [formData, setFormData] = useState(initialState);
const [errors, setErrors] = useState({});
const handleChange = useCallback((field, value) => {
setFormData(prev => ({
...prev,
[field]: value
}));
}, []);
const validateField = useCallback((field, value) => {
const validationRules = {
email: /^[^\s@]+@[^\s@]+\.[^\s@]+$/,
phone: /^\+?[\d\s-]{10,}$/
};
if (validationRules[field] && !validationRules[field].test(value)) {
setErrors(prev => ({
...prev,
[field]: `Invalid ${field} format`
}));
} else {
setErrors(prev => {
const { [field]: removed, ...rest } = prev;
return rest;
});
}
}, []);
return { formData, errors, handleChange, validateField };
}
This pattern keeps your form logic clean and easy to maintain while ensuring validation is handled efficiently.
Using Context for Data Management
The Context API is a great choice for managing data that needs to be shared across multiple components, even when deeply nested. Here’s how you can set up a context for managing theme and user preferences:
const PreferencesContext = createContext();
function PreferencesProvider({ children }) {
const [preferences, setPreferences] = useState({
theme: 'light',
language: 'en-US',
notifications: true
});
const updatePreference = useCallback((key, value) => {
setPreferences(prev => ({
...prev,
[key]: value
}));
}, []);
return (
<PreferencesContext.Provider value={{ preferences, updatePreference }}>
{children}
</PreferencesContext.Provider>
);
}
This approach simplifies state sharing and makes your components more modular and easier to test.
UXPin for React Prototyping
UXPin takes prototyping to the next level by enabling real-time data binding with React components. This means you can create interactive components that respond instantly to user input and state changes.
// Example of a UXPin-ready React component with data binding
function UserProfile({ userData, onUpdate }) {
return (
<div className="profile-container">
<input
type="text"
value={userData.name}
onChange={(e) => onUpdate('name', e.target.value)}
className="profile-input"
/>
<select
value={userData.theme}
onChange={(e) => onUpdate('theme', e.target.value)}
className="theme-selector"
>
<option value="light">Light Theme</option>
<option value="dark">Dark Theme</option>
</select>
</div>
);
}
UXPin integrates seamlessly with libraries like MUI and Ant Design, letting you prototype complex, interactive interfaces that closely mimic your final product. Its AI Component Creator even generates React components directly from your designs.
Some standout features of UXPin include:
- Real-time state management
- Conditional rendering
- Dynamic form handling with validation
- Interactive data visualizations
- Custom event handling
These tools make it easier to bridge the gap between design and development, saving time and improving collaboration.
Summary
Dynamic data binding in React is a key element for creating responsive and interactive user interfaces. It relies on a one-way data flow – from parent to child components – and effective state management to ensure components update instantly as data changes.
The foundation of successful data binding is React’s predictable one-way data flow. Information moves from parent to child components, and managing state properly helps avoid unnecessary re-renders and inconsistencies.
Here are some practical tips to keep in mind:
- State Management: Keep state close to where it’s used, lifting it up only when sharing between components is necessary.
- Event Handling: Use clear and predictable methods to manage user interactions and update data.
- Component Communication: Pass information efficiently between parent and child components using props and callbacks.
- Performance Optimization: Reduce unnecessary renders by applying techniques like memoization and structuring components thoughtfully.
These practices allow your React components to update dynamically, forming the backbone of interactive interfaces.
Tools like UXPin further simplify the process by enabling real-time data binding with code-backed components. This makes it easier to design, build, and test interactive features, streamlining development and improving user experiences.
Strong data binding practices lead to scalable and maintainable architectures that can evolve with your application’s growth.